本文共 203 字,大约阅读时间需要 1 分钟。
推荐适合学生党做深度学习使用GPU的平台(薅羊毛)
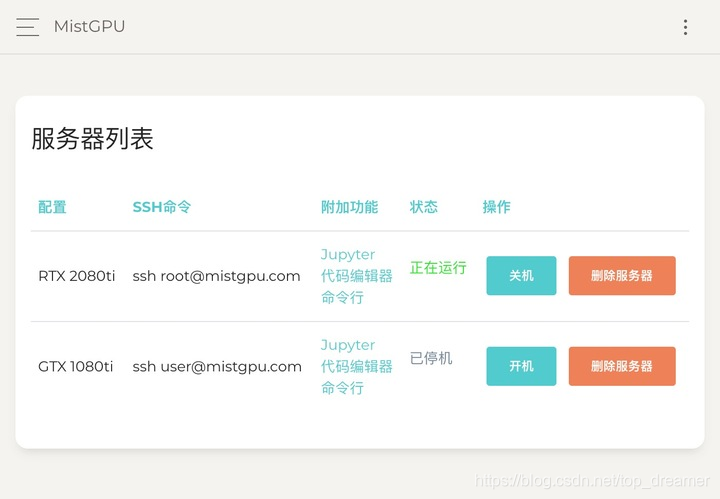
如果小伙伴在实验室一块GPU都没有怎么做深度学习?那我推荐下MistGPU平台,使用特别方便,传输文件速度也特别快(存储文件1G以下免费),可以按需使用,使用量比较大的也可以联系客服按月使用。我目前在使用ing!注册就有免费试用,每邀请一个用户也有赠送使用8元金额,无上限!欢迎一起薅羊毛(链接如下)~
截图展示我目前正在使用的gtx1080ti的机器:
转载地址:http://vogs.baihongyu.com/